Comprehensive Guide to CI/CD in iOS Using Swift
- Abhishek Bagela
- Jan 8
- 4 min read
Continuous Integration (CI) and Continuous Deployment/Delivery (CD) are essential practices in modern software development. They streamline the development workflow by automating code integration, testing, and deployment, ensuring faster delivery of high-quality applications. In this guide, we’ll explore CI/CD concepts, their significance in iOS development, and how to set up a CI/CD pipeline using Swift.
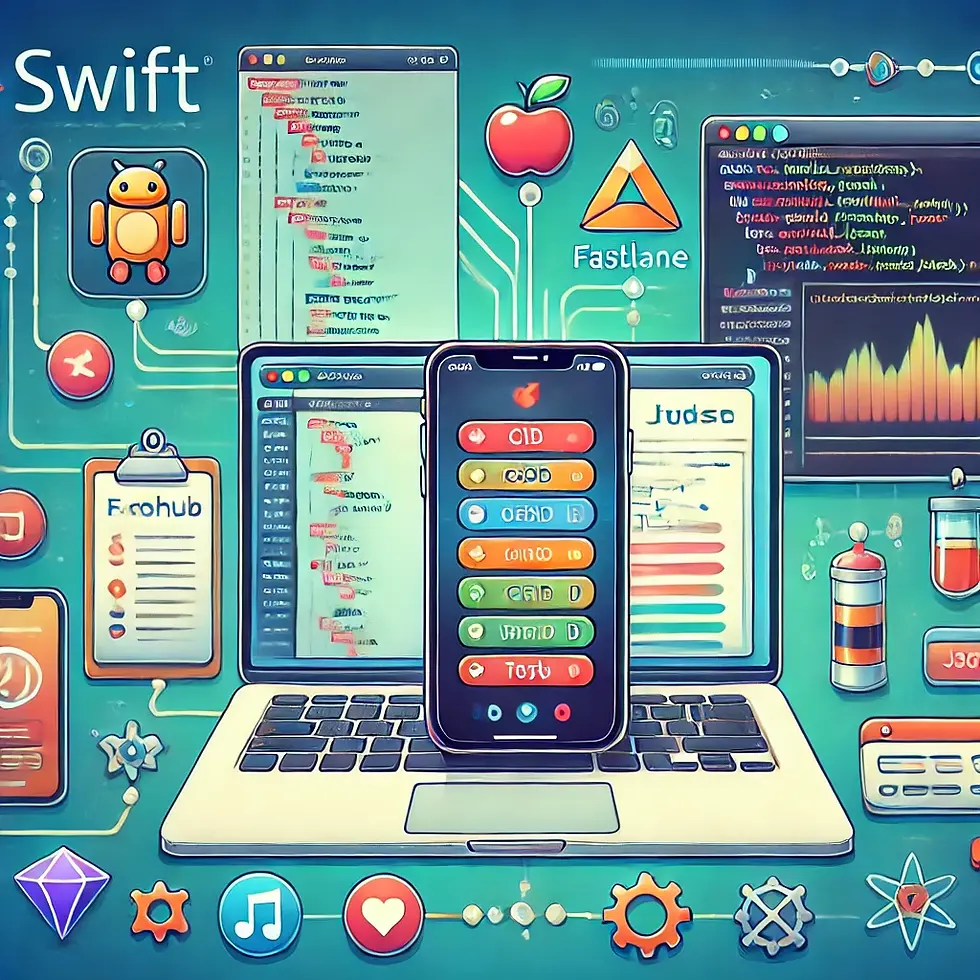
1. What is CI/CD?
• Continuous Integration (CI): Automates the process of merging code changes from multiple contributors into a shared repository. It involves automated builds and tests to identify issues early.
• Continuous Delivery (CD): Ensures the application is always in a deployable state. While deployment is manual, the process is automated up to the staging environment.
• Continuous Deployment (CD): Extends Continuous Delivery by automatically deploying to production after passing tests.
2. Importance of CI/CD in iOS Development
1. Faster Development Cycles: Automates repetitive tasks like building, testing, and deploying apps.
2. Improved Code Quality: Early detection of bugs and issues through automated testing.
3. Consistency: Ensures uniform builds across all team members.
4. Efficient Collaboration: Teams can focus on writing code rather than managing builds.
3. Key Components of CI/CD
3.1 Version Control System (VCS)
• Stores the source code and tracks changes.
• Popular choices: Git, GitHub, GitLab, Bitbucket.
3.2 Build Automation
• Automates app compilation and packaging.
• For iOS: Use xcodebuild or Fastlane.
3.3 Automated Testing
• Runs unit tests, UI tests, and integration tests automatically.
• Frameworks: XCTest, Quick, Nimble.
3.4 Deployment Automation
• Automates app distribution to testers or App Store.
• Tools: Fastlane, TestFlight.
4. Setting Up CI/CD for an iOS App
4.1 Prerequisites
• A working iOS app written in Swift.
• Access to a VCS (e.g., GitHub).
• A CI/CD tool like GitHub Actions, Bitrise, or Jenkins.
4.2 Step 1: Configure Git Repository
Push your project to a Git repository.
bash
git init
git add .
git commit -m "Initial commit"
git remote add origin <repository-url>
git push -u origin main
4.3 Step 2: Use Fastlane for Automation
Fastlane simplifies build, testing, and deployment tasks.
Install Fastlane
bash
sudo gem install fastlane -NV
cd <your-project-folder>
fastlane init
Fastlane Configuration Example (Fastfile)
ruby
default_platform(:ios)
platform :ios do
desc "Run tests"
lane :test do
scan
end
desc "Build app"
lane :build do
gym
end
desc "Distribute app via TestFlight"
lane :beta do
build
pilot
end
end
Run a lane using:
bash
fastlane test
4.4 Step 3: Set Up a CI Tool
GitHub Actions Example
Create a .github/workflows/ci.yml file.
yaml
name: iOS CI/CD Pipeline
on:
push:
branches:
- main
pull_request:
branches:
- main
jobs:
build:
runs-on: macos-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Install dependencies
run: bundle install
- name: Run tests
run: fastlane test
- name: Build app
run: fastlane build
- name: Deploy to TestFlight
run: fastlane beta
Bitrise
1. Create an account on Bitrise.
2. Add your project and configure workflows using their UI.
3. Add steps like Xcode Build, Xcode Test, and Deploy to App Store.
4.5 Step 4: Automated Testing with XCTest
Write unit and UI tests to ensure code reliability.
Unit Test Example
swift
import XCTest
class MyAppTests: XCTestCase {
func testExample() {
let value = 2 + 2
XCTAssertEqual(value, 4, "Math works!")
}
}
UI Test Example
swift
import XCTest
class MyAppUITests: XCTestCase {
func testButtonTap() {
let app = XCUIApplication()
app.launch()
app.buttons["MyButton"].tap()
XCTAssertTrue(app.staticTexts["ButtonTapped"].exists)
}
}
5. Advanced CI/CD Practices
5.1 Caching
Reduce build times by caching dependencies.
Example (GitHub Actions):
yaml
- name: Cache CocoaPods
uses: actions/cache@v3
with:
path: Pods
key: ${{ runner.os }}-pods-${{ hashFiles('Podfile.lock') }}
restore-keys: |
${{ runner.os }}-pods-
5.2 Parallel Testing
Distribute tests across multiple machines to speed up execution.
6. Challenges and Solutions
Challenge | Solution |
Code Signing Issues | Use a CI/CD tool’s keychain for certificates. |
Long Build Times | Use caching and parallelization. |
Managing Dependencies | Use dependency managers like CocoaPods or Swift Package Manager. |
Integration of New Tools | Gradually introduce and test new tools. |
7. Benefits of CI/CD in iOS Development
1. Scalability: CI/CD pipelines can easily scale with your team.
2. Improved Feedback Loop: Get immediate feedback on code changes.
3. Reduced Manual Effort: Automates repetitive tasks, saving time.
4. Enhanced Collaboration: Encourages a collaborative and iterative development process.
8. Example CI/CD Workflow for an iOS App
1. Push Changes: Developer pushes changes to the Git repository.
2. Run CI Pipeline:
• Code is checked out.
• Dependencies are installed.
• Unit and UI tests are executed.
3. Build and Deploy:
• App is built using xcodebuild.
• Uploaded to TestFlight or the App Store.
9. Tools for CI/CD in iOS
Tool | Use Case | Features |
GitHub Actions | Git-based CI/CD workflows | Easy integration with GitHub. |
Bitrise | CI/CD for mobile apps | Pre-built workflows for iOS. |
Jenkins | Open-source automation | Highly customizable. |
CircleCI | Continuous integration | Fast build times. |
10. Conclusion
CI/CD in iOS development using Swift is a game-changer for delivering high-quality apps efficiently. By automating testing, building, and deployment processes, you ensure that your app remains robust and ready for release at any time. With tools like Fastlane, GitHub Actions, and Bitrise, setting up a CI/CD pipeline has never been easier.
Start implementing CI/CD in your projects today to enhance your development workflow and accelerate your app’s success! 🚀
Comments