In-Depth Article on Objective-C Language
- Abhishek Bagela
- Jan 13
- 3 min read
Objective-C is a powerful, object-oriented programming language primarily used for macOS and iOS app development. It builds upon the C programming language by adding object-oriented capabilities and dynamic runtime features, making it flexible and dynamic for application development. Below is a detailed exploration of Objective-C:
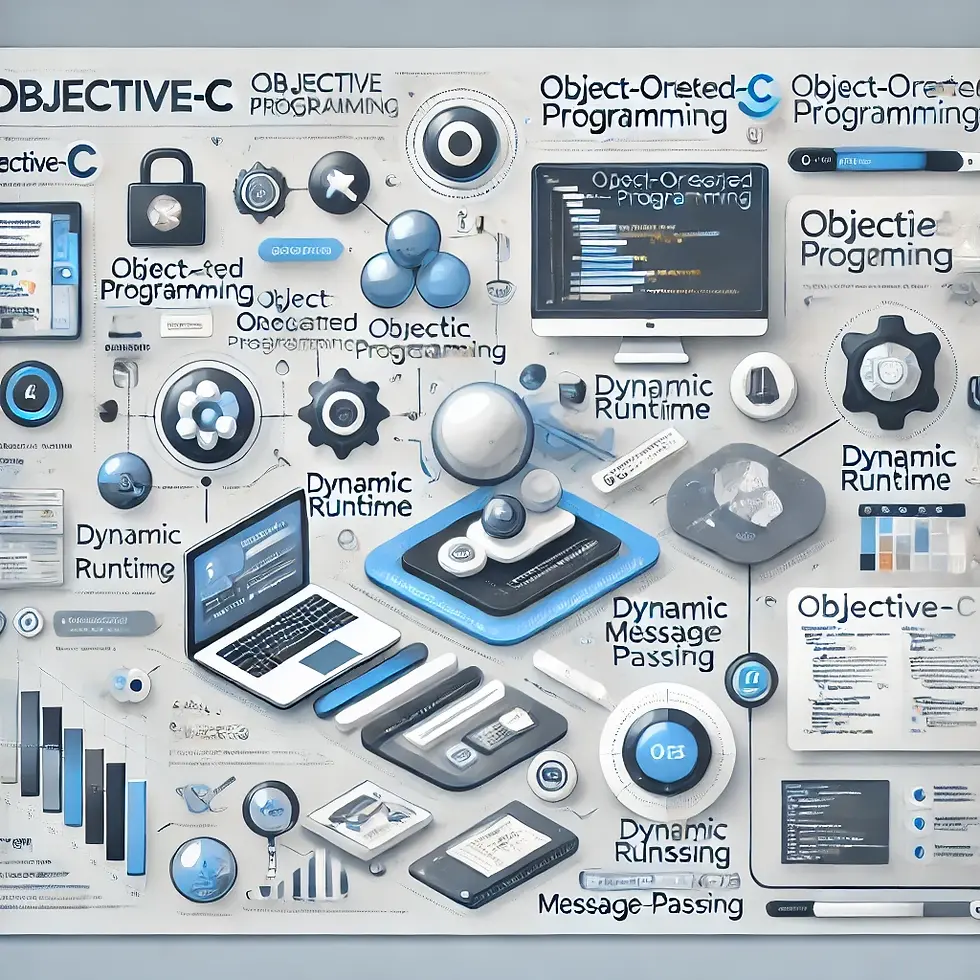
1. History and Overview
• Developed by: Brad Cox and Tom Love in the early 1980s.
• Purpose: To provide object-oriented capabilities to the C language.
• Adopted by Apple: Became the primary language for macOS and iOS development after Apple acquired NeXT in the late 1990s.
• Syntax: A blend of C and Smalltalk, featuring message-passing syntax.
2. Key Features
1. Object-Oriented:
• Supports classes, objects, inheritance, polymorphism, and encapsulation.
• Example:
@interface Car : NSObject
@property NSString *model;
- (void)drive;
@end
@implementation Car
- (void)drive {
NSLog(@"Driving a %@", self.model);
}
@end
2. Dynamic Runtime:
• Objective-C defers many decisions to runtime, making it highly flexible.
• Methods and properties can be added or removed at runtime.
3. Message Passing:
• Unlike direct method calls in other languages, Objective-C uses a Smalltalk-style message-passing approach.
• Example:
[object methodName];
4. Categories:
• Allows adding methods to existing classes without modifying their original implementation.
• Example:
@interface NSString (Reversed)
- (NSString *)reversedString;
@end
@implementation NSString (Reversed)
- (NSString *)reversedString {
NSUInteger length = [self length];
NSMutableString *reversed = [NSMutableString stringWithCapacity:length];
for (NSInteger i = length - 1; i >= 0; i--) {
[reversed appendFormat:@"%C", [self characterAtIndex:i]];
}
return reversed;
}
@end
5. Protocols:
• Similar to interfaces in other languages, they define a blueprint of methods that conforming classes must implement.
• Example:
@protocol Drivable
- (void)start;
- (void)stop;
@end
6. Memory Management:
• Initially used manual retain-release model (retain, release, autorelease).
• Later replaced by Automatic Reference Counting (ARC).
7. Interoperability with C and C++:
• Allows integration of legacy C or C++ code with Objective-C.
3. Basic Syntax
1. Class Declaration and Implementation:
• Declaration:
@interface MyClass : NSObject
- (void)myMethod;
@end
• Implementation:
@implementation MyClass
- (void)myMethod {
NSLog(@"Hello, Objective-C!");
}
@end
2. Properties:
• Simplify getter and setter method creation.
• Example:
@property NSString *name;
3. Memory Management:
• Example with ARC:
@property (strong, nonatomic) NSString *title;
4. Objective-C vs Swift
Feature | Objective-C | Swift |
Syntax Complexity | Verbose and C-like | Simplified and modern |
Memory Management | Automatic (ARC) | |
Performance | Slightly slower | Optimized for speed |
Dynamic Features | Highly dynamic | Static and safe |
Interoperability | Seamless with C | Seamless with Objective-C |
5. Advanced Concepts
1. Blocks:
• Objective-C’s way of handling closures.
• Example:
int (^sumBlock)(int, int) = ^(int a, int b) {
return a + b;
};
NSLog(@"Sum: %d", sumBlock(3, 5));
2. Selectors:
• Represent method names.
• Example:
SEL mySelector = @selector(myMethod);
[object performSelector:mySelector];
3. Dynamic Typing:
• Use of id for generic object handling.
• Example:
id dynamicObject = @"This is a string";
NSLog(@"%@", dynamicObject);
6. Practical Examples
1. Simple Hello World:
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSLog(@"Hello, Objective-C!");
}
return 0;
}
2. Custom Class:
@interface Animal : NSObject
@property NSString *name;
- (void)speak;
@end
@implementation Animal
- (void)speak {
NSLog(@"%@ says Hello!", self.name);
}
@end
int main() {
Animal *dog = [[Animal alloc] init];
dog.name = @"Dog";
[dog speak];
return 0;
}
3. Delegate Pattern:
@protocol PrinterDelegate
- (void)printerDidFinishPrinting;
@end
@interface Printer : NSObject
@property (weak) id<PrinterDelegate> delegate;
- (void)printDocument;
@end
@implementation Printer
- (void)printDocument {
NSLog(@"Printing...");
[self.delegate printerDidFinishPrinting];
}
@end
7. Pros and Cons
Pros:
• Mature and stable.
• Flexible with dynamic runtime.
• Interoperability with C/C++.
Cons:
• Verbose syntax.
• Steep learning curve compared to Swift.
• Less future-proof as Apple prefers Swift.
8. Conclusion
While Objective-C has been largely replaced by Swift for new development, it remains an important language in the Apple ecosystem, especially for maintaining legacy code. Its unique dynamic runtime and message-passing style provide a solid foundation for building robust applications.
Comments