In-Depth Guide to UIKit in iOS Using Swift
- Abhishek Bagela
- Jan 8
- 4 min read
UIKit is the foundational framework for building user interfaces in iOS applications. It provides a comprehensive set of tools and classes for creating, managing, and interacting with views, view controllers, gestures, animations, and more. UIKit has been a core part of iOS development since its inception, and it remains a critical skill for iOS developers.
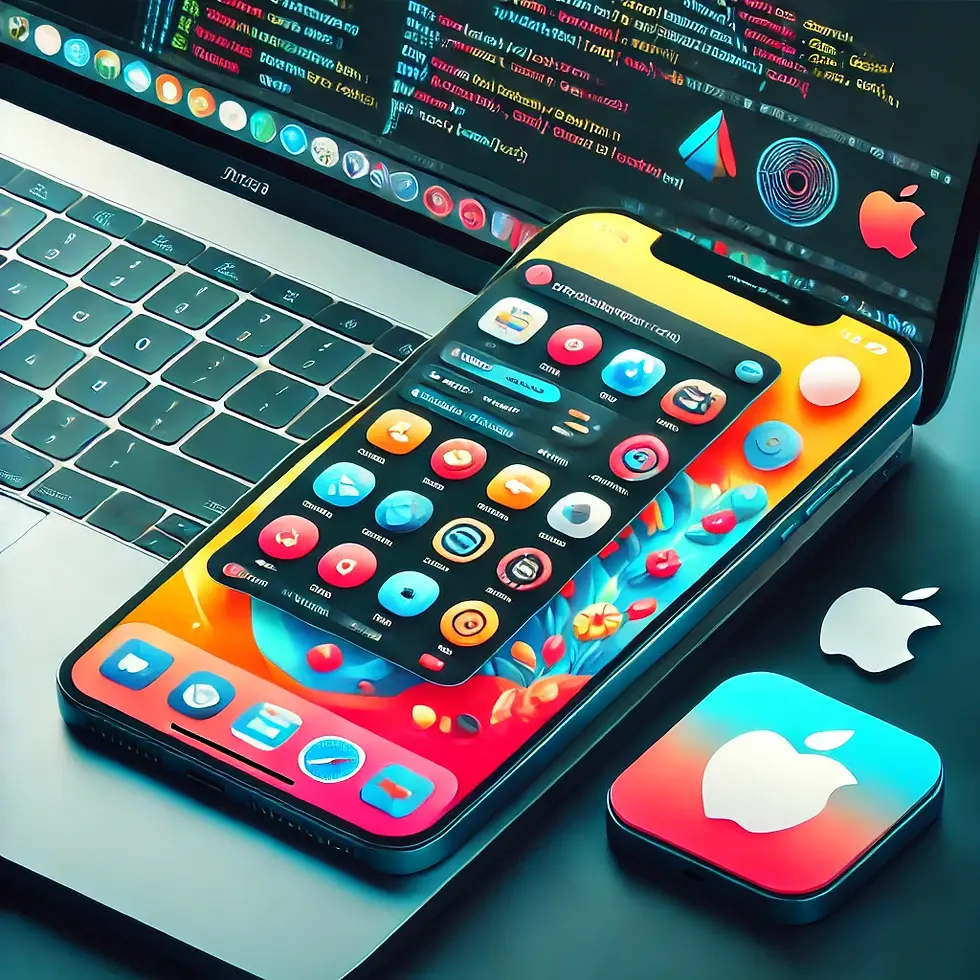
1. What is UIKit?
UIKit is an Apple framework designed to help developers create intuitive and interactive user interfaces for iOS and iPadOS applications. It works in harmony with the Cocoa Touch framework, enabling a wide range of functionalities, from basic UI controls to complex animations.
Key Features of UIKit:
• Building user interfaces programmatically or with Interface Builder.
• Managing the app’s lifecycle and navigation flow.
• Handling user interactions, gestures, and animations.
• Managing text, images, and media content.
2. Structure of UIKit
2.1 Views and View Hierarchy
• UIView: The fundamental building block of any user interface in UIKit.
• View Hierarchy: Views are organized in a tree structure, with a root view containing subviews.
Example:
let rootView = UIView(frame: CGRect(x: 0, y: 0, width: 300, height: 300))
rootView.backgroundColor = .white
let subView = UIView(frame: CGRect(x: 50, y: 50, width: 200, height: 200))
subView.backgroundColor = .blue
rootView.addSubview(subView)
2.2 View Controllers
• UIViewController: Manages a single screen in the app.
• Responsible for handling view loading, user interactions, and navigation.
Example:
class MyViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .white
}
}
2.3 Navigation and Presentation
• UINavigationController: Manages a stack of view controllers to enable navigation.
• UITabBarController: Allows switching between multiple view controllers.
• Modal Presentation: Presents a view controller modally.
Example:
let navigationController = UINavigationController(rootViewController: MyViewController())
present(navigationController, animated: true)
2.4 Controls and Gestures
• UIControl: A base class for interactive elements like buttons and sliders.
• Gestures: UIKit provides gesture recognizers like UITapGestureRecognizer and UISwipeGestureRecognizer.
Example:
let button = UIButton(type: .system)
button.setTitle("Tap Me", for: .normal)
button.addTarget(self, action: #selector(buttonTapped), for: .touchUpInside)
@objc func buttonTapped() {
print("Button was tapped!")
}
2.5 Animations
UIKit makes it easy to create smooth animations using the UIView.animate method.
Example:
UIView.animate(withDuration: 1.0) {
self.view.alpha = 0.5
}
3. Essential UIKit Classes and Their Uses
Class | Description | Example |
UILabel | Displays a single line or multiline text. | let label = UILabel() |
UIButton | Represents a tappable button. | let button = UIButton(type: .system) |
UIImageView | Displays an image. | let imageView = UIImageView(image: UIImage(named: "image")) |
UITableView | Displays a scrollable list of items. | Customizable for complex lists. |
UICollectionView | Similar to UITableView, but supports custom layouts. | Perfect for grids and custom item layouts. |
UITextField | Provides a text input field. | Useful for forms or search bars. |
UIScrollView | Allows scrolling through content larger than the visible area. | let scrollView = UIScrollView() |
4. Building a Simple UIKit App in Swift
Here’s an example of a simple UIKit app with a button and a label.
Step 1: Create a View Controller
import UIKit
class ViewController: UIViewController {
private let label = UILabel()
private let button = UIButton(type: .system)
override func viewDidLoad() {
super.viewDidLoad()
view.backgroundColor = .white
setupLabel()
setupButton()
}
private func setupLabel() {
label.text = "Hello, UIKit!"
label.textAlignment = .center
label.frame = CGRect(x: 50, y: 200, width: 300, height: 50)
view.addSubview(label)
}
private func setupButton() {
button.setTitle("Press Me", for: .normal)
button.frame = CGRect(x: 100, y: 300, width: 200, height: 50)
button.addTarget(self, action: #selector(buttonPressed), for: .touchUpInside)
view.addSubview(button)
}
@objc private func buttonPressed() {
label.text = "Button Pressed!"
}
}
Step 2: Set the Root View Controller
Update the SceneDelegate to make ViewController the root view controller.
import UIKit
class SceneDelegate: UIResponder, UIWindowSceneDelegate {
var window: UIWindow?
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
guard let windowScene = (scene as? UIWindowScene) else { return }
let window = UIWindow(windowScene: windowScene)
window.rootViewController = ViewController()
self.window = window
window.makeKeyAndVisible()
}
}
5. Advantages of Using UIKit
1. Mature Framework: Time-tested and stable.
2. Highly Customizable: Supports both basic and advanced UI designs.
3. Widespread Community Support: Tons of resources, tutorials, and third-party libraries.
4. Rich Set of Components: From simple labels to complex table views.
6. Limitations of UIKit
• Declarative UI: Lacks the declarative approach of SwiftUI.
• Steep Learning Curve: Complex for beginners when creating advanced UIs.
• Compatibility: Requires more manual work to support multiple devices.
7. UIKit vs. SwiftUI
Feature | UIKit | SwiftUI |
Approach | Imperative | Declarative |
Learning Curve | Moderate to High | Low |
Customizability | Extremely high | Good but improving |
Compatibility | Works with older iOS versions | Requires iOS 13+ |
8. Career Relevance of UIKit
UIKit is essential for:
• Legacy app development and maintenance.
• Building apps for companies with large, existing codebases.
• Creating highly customized user interfaces.
Even with the rise of SwiftUI, UIKit expertise remains valuable as many apps continue to use it.
9. Conclusion
UIKit is a cornerstone of iOS development, offering flexibility, power, and a rich set of features. While SwiftUI is gaining popularity, mastering UIKit is essential for any iOS developer aiming to work on real-world applications. Use this guide as a foundation to explore the limitless possibilities of UIKit in your projects!
Happy coding! 🚀
Comments