SwiftUI has transformed how developers build user interfaces for Apple platforms by simplifying the process. With its clean and cohesive layout system, SwiftUI makes it easy for beginners to create visually appealing and functional applications. This guide will equip you with a solid understanding of the SwiftUI layout system, supported by practical examples and essential tips.
Understanding SwiftUI Layout System
At its core, SwiftUI uses a declarative approach. This means you describe what your UI should look like and let SwiftUI handle the layout. Instead of manually managing constraints, you define your elements—known as views—which combine to create intricate interfaces.
Key components of the SwiftUI layout system include:
Views: Basic elements such as `Text`, `Image`, and `Button` that display content.
Stacks: Containers like `HStack`, `VStack`, and `ZStack` that help arrange views in various ways (horizontally, vertically, or layered).
Modifiers: These are methods you can apply to views to change their appearance or behavior.
GeometryReader: This view provides size and position details necessary for its child views.
Together, these elements create a cohesive layout that adapts to different device sizes and orientation.
Creating Layouts with Stacks
SwiftUI's stacking concepts make layout creation straightforward. Here’s how each stack functions with practical examples.
VStack
A `VStack` arranges views in a vertical stack. For instance:
```swift
VStack {
Text("Hello, World!")
.font(.largeTitle)
Text("Welcome to SwiftUI.")
.font(.subheadline)
}
```
In this example, two text views are stacked vertically, centered on the screen.
HStack
An `HStack` places views in a horizontal line. For example:
```swift
HStack {
Image(systemName: "star.fill")
Text("Favorites")
.font(.headline)
}
```
This code displays a star icon alongside the word "Favorites," creating a visually appealing button-like element.
ZStack
A `ZStack` overlays its children on top of each other. This is particularly useful for designs like badges:
```swift
ZStack {
Circle()
.fill(Color.blue)
.frame(width: 100, height: 100)
Text("1")
.foregroundColor(.white)
}
```
The example creates a blue circle with a "1" label in the center, great for notifications.
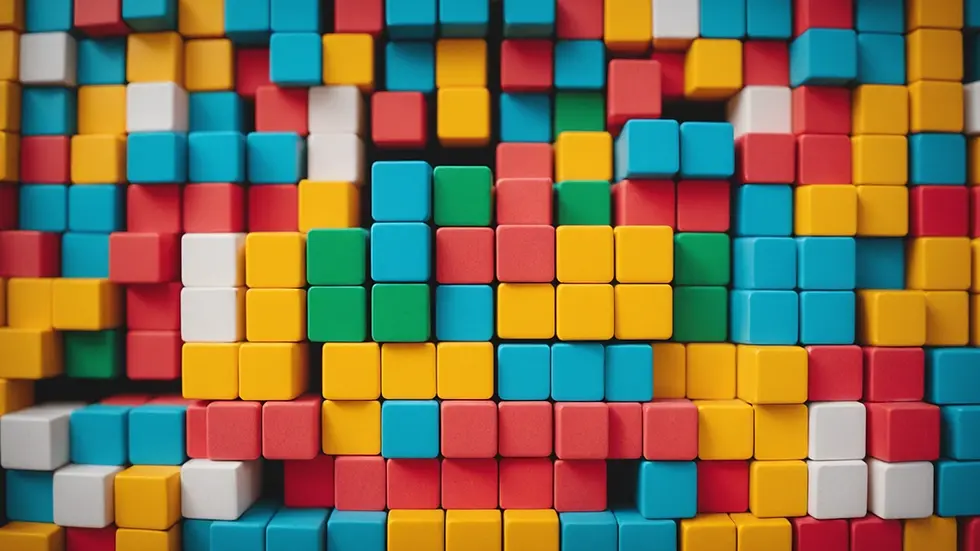
Layout Modifiers
Modifiers provide a way to enhance your views. They help change the layout by adjusting appearance or behavior. Here are some common modifiers and their uses:
Padding
Use padding to create space around content. For example:
```swift
Text("Padded Text")
.padding()
.background(Color.yellow)
```
This adds yellow background padding around the text, improving visibility.
Alignment
Align content within stacks using alignment modifiers. For example:
```swift
HStack(alignment: .top) {
Text("Top")
Text("Bottom")
.alignmentGuide(.top) { d in d[.bottom] }
}
```
Here, the second text aligns to the top of the first, allowing for more precise control over layout.
Backgrounds
Adding backgrounds enhances the viewer's experience:
```swift
Text("With Background")
.padding()
.background(Color.green)
```
A green background helps the text stand out and adds an engaging visual element.
Combining these modifiers can create intricate designs without much complexity in code. For example, combining padding and background can create buttons that are both functional and attractive.
Key Takeaways
Embrace the Declarative Syntax: SwiftUI's declarative nature means you simply state what you want. This leads to efficient coding and quick updates.
Experiment with Stacks: Use stacks to minimize repetitive code. Each stack type has a unique purpose, so choose the one that fits your needs best.
Use Modifiers Wisely: Familiarize yourself with modifiers like padding, background, and alignment to create stunning layouts effortlessly.
Adaptive Layouts: SwiftUI effortlessly adapts to various screen sizes and orientations. Use `.frame()` and `GeometryReader` when specific positioning is necessary.
Previewing: Regularly utilize the SwiftUI preview feature in Xcode. This aids in quickly identifying and correcting layout issues.
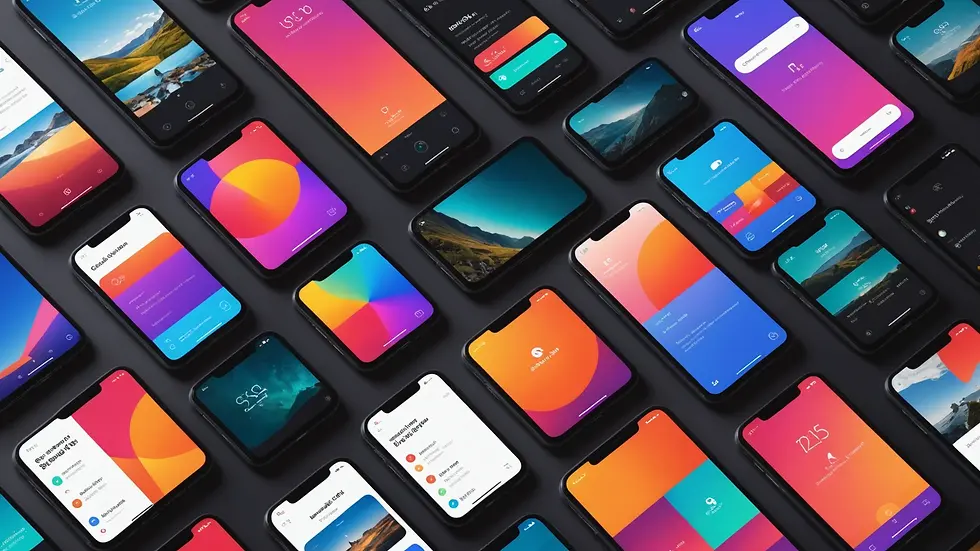
Your SwiftUI Journey Begins Here
Understanding the SwiftUI layout system is a game changer for developers. By mastering the basics, you set yourself up for success in crafting rich user interfaces. As you begin developing your applications, be sure to explore SwiftUI's documentation and connect with community resources. SwiftUI is constantly evolving, making it essential to stay updated with new features and improvements. Happy coding!
Comments