Swift Programming Language: A Full Tutorial with Examples and Definitions
- Abhishek Bagela
- Jan 6
- 3 min read
Swift is a powerful and user-friendly programming language developed by Apple for iOS, macOS, watchOS, and tvOS development. This tutorial provides a comprehensive introduction to Swift, with examples and definitions to help you grasp its core concepts.
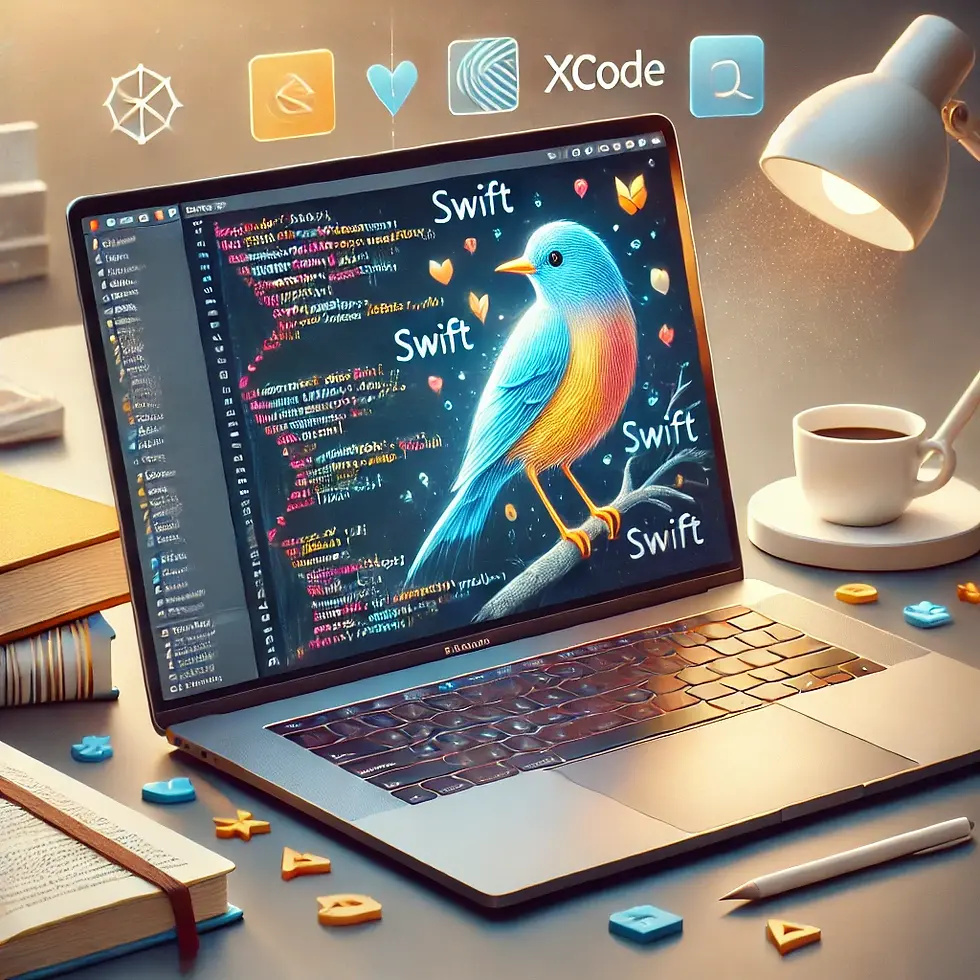
1. Introduction to Swift
Swift is a general-purpose, high-level programming language known for its:
• Safety: Eliminates common programming errors with strict type checking and optionals.
• Performance: Built on LLVM for optimized performance.
• Modern Syntax: Easy to read and write.
2. Setting Up Swift
1. Install Xcode:
Download Xcode from the Mac App Store. It includes everything you need to write, test, and debug Swift code.
2. Use Swift Playgrounds:
Swift Playgrounds in Xcode is a great way to experiment with code interactively.
3. Basic Syntax
3.1 Variables and Constants
• Variables: Mutable values declared using var.
• Constants: Immutable values declared using let.
var name = "John" // Mutable
let age = 25 // Immutable
name = "Doe" // Allowed
// age = 30 // Error: Cannot assign to a constant
3.2 Data Types
Swift is strongly typed, meaning you must define the type of variable if it’s not inferable.
var integer: Int = 10
var double: Double = 20.5
var string: String = "Hello, Swift!"
var boolean: Bool = true
4. Control Flow
4.1 Conditionals
let score = 85
if score > 90 {
print("A grade")
} else if score > 75 {
print("B grade")
} else {
print("C grade")
}
4.2 Loops
// For loop
for i in 1...5 {
print(i)
}
// While loop
var count = 0
while count < 3 {
print(count)
count += 1
}
// Repeat-while loop
repeat {
print(count)
count -= 1
} while count > 0
5. Functions
Functions encapsulate reusable code.
5.1 Defining Functions
func greet(name: String) -> String {
return "Hello, \(name)!"
}
print(greet(name: "Alice")) // Output: Hello, Alice!
5.2 Parameter Defaults
func greet(name: String = "Guest") {
print("Hello, \(name)!")
}
greet() // Output: Hello, Guest!
greet(name: "Bob") // Output: Hello, Bob!
6. Optionals
Optionals handle the absence of a value.
6.1 Declaring Optionals
var optionalString: String? = nil
if let unwrapped = optionalString {
print("Value: \(unwrapped)")
} else {
print("No value")
}
6.2 Force Unwrapping
optionalString = "Swift"
print(optionalString!) // Unsafe if nil, but outputs: Swift
7. Collections
7.1 Arrays
Ordered collections of elements.
var fruits = ["Apple", "Banana", "Cherry"]
fruits.append("Mango")
print(fruits[0]) // Output: Apple
7.2 Dictionaries
Key-value pairs.
var capitals = ["India": "Delhi", "USA": "Washington"]
capitals["France"] = "Paris"
print(capitals["India"]!) // Output: Delhi
8. Object-Oriented Programming
8.1 Classes
Blueprints for creating objects.
class Person {
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func greet() {
print("Hi, I'm \(name) and I'm \(age) years old.")
}
}
let person = Person(name: "Alice", age: 25)
person.greet()
8.2 Inheritance
class Employee: Person {
var jobTitle: String
init(name: String, age: Int, jobTitle: String) {
self.jobTitle = jobTitle
super.init(name: name, age: age)
}
override func greet() {
print("Hi, I'm \(name), a \(jobTitle).")
}
}
let employee = Employee(name: "Bob", age: 30, jobTitle: "Developer")
employee.greet()
9. Protocol-Oriented Programming
9.1 Protocols
Protocols define a blueprint for methods and properties.
protocol Describable {
func describe() -> String
}
class Car: Describable {
var model: String
init(model: String) {
self.model = model
}
func describe() -> String {
return "Car model: \(model)"
}
}
let car = Car(model: "Tesla")
print(car.describe())
10. Advanced Topics
10.1 Closures
Closures are self-contained blocks of functionality.
let multiply = { (a: Int, b: Int) -> Int in
return a * b
}
print(multiply(3, 4)) // Output: 12
10.2 Generics
Generics enable reusable, type-safe functions and classes.
func swapValues<T>(a: inout T, b: inout T) {
let temp = a
a = b
b = temp
}
var x = 10, y = 20
swapValues(a: &x, b: &y)
print("x: \(x), y: \(y)") // Output: x: 20, y: 10
12. Conclusion
Swift is a versatile and modern programming language ideal for iOS application development. With its safety, readability, and performance, mastering Swift opens doors to a fulfilling career in app development. Start experimenting with Swift Playgrounds and building your first app today! 🚀
Comentarios